Plotting Soundings with Observed ACARS data
[1]:
# first, import sounderpy! Its fun to import as 'spy'!
import sounderpy as spy
## ---------------------------------- SOUNDERPY ----------------------------------- ##
## Vertical Profile Data Retrieval and Analysis Tool For Python ##
## v3.0.3 | Mar. 2024 | (C) Kyle J Gillett ##
## Docs: https://kylejgillett.github.io/sounderpy/ ##
## --------------------- THANK YOU FOR USING THIS PACKAGE! ------------------------ ##
Understanding SounderPy Functionality
To get ACARS data, we need to use the ``acars_data()`` class
Reading the documentation on ACARS data access may prove useful before using this notebook: https://kylejgillett.github.io/sounderpy/gettingdata.html#observed-data-acars-aircraft-obs
[10]:
# First we need to set up a 'connection' to the ACARS data.
# We do this by creating a variable called `acars_conn` which is set equal
# to the `acars_data()` class which needs a year, month, day and hour as strings.
# Lets look for flights on Dec. 30th 2023 at 12 UTC
acars_conn = spy.acars_data('2024', '02', '28', '01')
# Now that we have the connection established, lets look for a profile
# to plot. We can do this by using the `.list_profiles() function:
acars_conn.list_profiles()
> LIST ACARS PROFILES FUNCTION --
---------------------------------
> AVAILABLE ACARS PROFILES FOR 2024-02-28 01Z...
> COMPLETE --------
> RUNTIME: 00:00:00
[10]:
['ATL_0120',
'ATL_0140',
'AUS_0130',
'AUS_0140',
'BNA_0110',
'BNA_0140',
'BOI_0130',
'BWI_0120',
'CHS_0100',
'CMH_0150',
'DAL_0100',
'DAL_0130',
'DTW_0100',
'FLL_0140',
'HDN_0120',
'HOU_0130',
'HOU_0140',
'IND_0100',
'JAN_0120',
'JAX_0110',
'LAS_0130',
'LAS_0150',
'LAX_0110',
'LAX_0130',
'LGA_0140',
'LGB_0120',
'MCO_0110',
'MCO_0150',
'MDW_0150',
'MFE_0140',
'MIA_0120',
'MSY_0120',
'OKC_0100',
'PHX_0120',
'PHX_0150',
'SAN_0100',
'SAT_0110',
'SAT_0140',
'SBA_0110',
'SJC_0130',
'SLC_0140',
'TPA_0100',
'TPA_0110']
[11]:
# These are all the available flights we can get data from across the ACARS network
# on Dec. 30th 2023 at 12 UTC. Now we can pick one and add it to the function
# `.get_profile()` to actually get the data. Lets try 'DAL_1210':
clean_data = acars_conn.get_profile('MDW_0150')
> ACARS DATA ACCESS FUNCTION --
---------------------------------
> COMPLETE --------
> RUNTIME: 00:00:00
> SUMMARY: 01:48Z Flight from MDW, Chicago Midway International Airport at 02-28-2024-01:48Z
> THERMODYNAMICS ---------------------------------------------
--- SBCAPE: 161.5 | MUCAPE: 266.5 | MLCAPE: 174.8 | MUECAPE: --
--- MU 0-3: 203.6 | MU 0-6: -- | SB 0-3: 114.6 | SB 0-6: --
> KINEMATICS -------------------------------------------------
--- 0-500 SRW: -- | 0-500 SWV: -- | 0-500 SHEAR: 31.7 | 0-500 SRH: --
--- 1-3km SRW: -- | 1-3km SWV: -- | 1-3km SHEAR: 14.9 | | 1-3km SRH: --
==============================================================
[12]:
# Lets check out the ACARS data that we just accessed
# and stored in the `clean_data` variable:
# `clean_data` is a python dictionary of 'clean' vertical profile data
# it comes with temperature, dewpoint, pressure, height, u and v
# as well as a number of useful 'meta data'
# note how these data only goes up to ~640 hPa
clean_data
[12]:
{'p': array([963.94, 957.16, 947.45, 936.69, 925.47, 915.02, 907.31, 902.15,
896.92, 891.81, 888.57, 886.84, 886.52, 886.41, 885.22, 882.64,
877.07, 861.79, 831.77, 798.73, 759.98, 715.57, 674.59, 643.88,
633.88]) <Unit('hectopascal')>,
'z': array([ 421., 480., 565., 660., 760., 854., 924., 971., 1019.,
1066., 1096., 1112., 1115., 1116., 1127., 1151., 1203., 1347.,
1636., 1964., 2363., 2841., 3304., 3666., 3787.]) <Unit('meter')>,
'T': array([19.05, 18.35, 17.75, 17.05, 16.85, 16.05, 15.05, 15.05, 15.25,
15.05, 15.05, 15.05, 15.05, 15.05, 15.05, 14.85, 14.85, 13.85,
12.35, 9.55, 6.05, 1.55, -2.75, -5.75, -6.95]) <Unit('degree_Celsius')>,
'Td': array([ 14.32, 14.21, 14.03, 13.81, 13.63, 13.48, 13.36, 13.34,
13.47, 13.4 , 13.4 , 13.3 , 13.28, 13.23, 13.2 , 13.06,
12.83, 11.72, 7.42, 1.42, 0.05, -2.35, -5.87, -10.96,
-11.29]) <Unit('degree_Celsius')>,
'u': array([-7.39615389, -8.69869788, -8.171829 , -6.28453171, -4.14735841,
-4.98239701, -1.97829915, 2.14054061, 6.85182957, 7.77943836,
9.85285618, 11.79727883, 11.79727883, 12.51350896, 12.51350896,
12.94781206, 14.33064401, 17.04226534, 20.45 , 26.36852438,
31.30372473, 35.69006064, 33.73703664, 26.52973621, 27.71686898]) <Unit('knot')>,
'v': array([ 6.42937848, 9.66088273, 16.03811744, 21.91676667, 29.50998845,
35.45159686, 37.74819641, 40.84394797, 43.26074932, 44.11938733,
42.67740884, 41.14200059, 41.14200059, 40.92984356, 40.92984356,
39.84926803, 39.37312081, 38.27755468, 35.42043901, 32.56241579,
33.5691349 , 33.28151997, 32.57947757, 28.44965899, 28.70165803]) <Unit('knot')>,
'site_info': {'site-id': 'MDW',
'site-name': 'Chicago Midway International Airport',
'site-lctn': 'United States',
'site-latlon': [41.79, -87.75],
'site-elv': '620',
'source': 'ACARS OBSERVED AIRCRAFT PROFILE',
'model': 'no-model',
'fcst-hour': 'no-fcst-hour',
'run-time': ['none', 'none', 'none', 'none'],
'valid-time': ['2024', '02', '28', '01:48']}}
LETS PLOT THE DATA ON A SOUNDING AND HODOGRAPH
[13]:
# lets make a sounding!
spy.build_sounding(clean_data)
> SOUNDING PLOTTER FUNCTION --
---------------------------------
> COMPLETE --------
> RUNTIME: 00:00:01
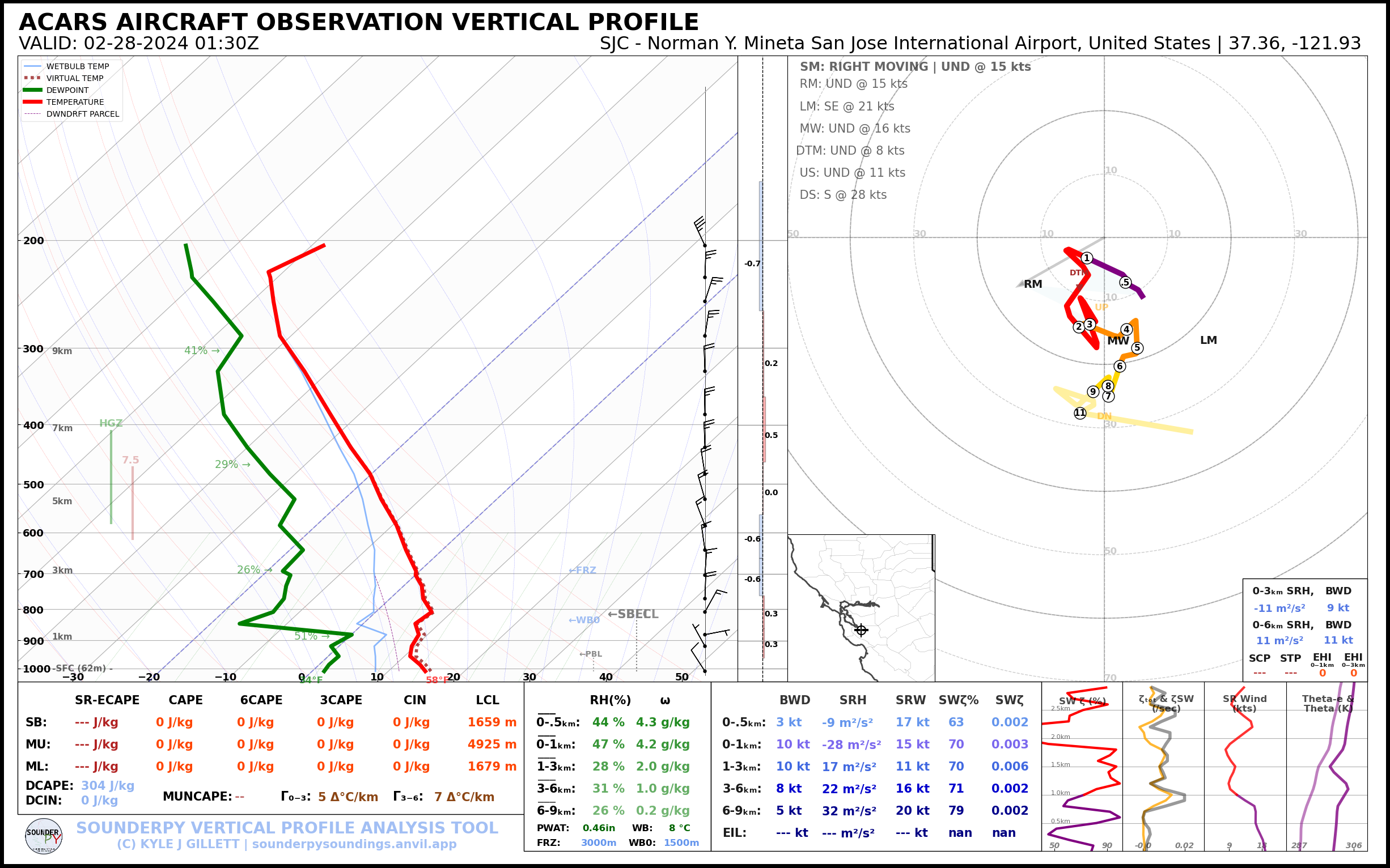
[ ]: